io.noties.markwon.Markwon Java Examples
The following examples show how to use
io.noties.markwon.Markwon.
You can vote up the ones you like or vote down the ones you don't like,
and go to the original project or source file by following the links above each example. You may check out the related API usage on the sidebar.
Example #1
Source File: Table.java From Markwon with Apache License 2.0 | 6 votes |
/** * Factory method to obtain an instance of {@link Table} * * @param markwon Markwon * @param tableBlock TableBlock to parse * @return parsed {@link Table} or null */ @Nullable public static Table parse(@NonNull Markwon markwon, @NonNull TableBlock tableBlock) { final Table table; final ParseVisitor visitor = new ParseVisitor(markwon); tableBlock.accept(visitor); final List<Row> rows = visitor.rows(); if (rows == null) { table = null; } else { table = new Table(rows); } return table; }
Example #2
Source File: InlineParserActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void pluginNoDefaults() { // a plugin with NO defaults registered final String md = "no [links](#) for **you** `code`!"; final Markwon markwon = Markwon.builder(this) // pass `MarkwonInlineParser.factoryBuilderNoDefaults()` no disable all .usePlugin(MarkwonInlineParserPlugin.create(MarkwonInlineParser.factoryBuilderNoDefaults())) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(MarkwonInlineParserPlugin.class, plugin -> { plugin.factoryBuilder() .addInlineProcessor(new BackticksInlineProcessor()); }); } }) .build(); markwon.setMarkdown(textView, md); }
Example #3
Source File: InlineParserActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void pluginWithDefaults() { // a plugin with defaults registered final String md = "no [links](#) for **you** `code`!"; final Markwon markwon = Markwon.builder(this) .usePlugin(MarkwonInlineParserPlugin.create()) // the same as: // .usePlugin(MarkwonInlineParserPlugin.create(MarkwonInlineParser.factoryBuilder())) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(MarkwonInlineParserPlugin.class, plugin -> { plugin.factoryBuilder() .excludeInlineProcessor(OpenBracketInlineProcessor.class); }); } }) .build(); markwon.setMarkdown(textView, md); }
Example #4
Source File: MainActivity.java From Markwon with Apache License 2.0 | 6 votes |
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // obtain an instance of Markwon // here we are creating as core markwon (no additional plugins are registered) final Markwon markwon = Markwon.create(this); final Adapt adapt = Adapt.create(); final List<Item> items = new ArrayList<>(); final SampleItem.OnClickListener onClickListener = this::showSample; for (Sample sample : Sample.values()) { items.add(new SampleItem(sample, markwon, onClickListener)); } adapt.setItems(items); final RecyclerView recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setHasFixedSize(true); recyclerView.addItemDecoration(createSampleItemDecoration()); recyclerView.setAdapter(adapt); }
Example #5
Source File: MarkwonEditorImplTest.java From Markwon with Apache License 2.0 | 6 votes |
@Test public void process() { // create markwon final Markwon markwon = Markwon.create(RuntimeEnvironment.application); // default punctuation final MarkwonEditor editor = MarkwonEditor.create(markwon); final SpannableStringBuilder builder = new SpannableStringBuilder("**bold**"); editor.process(builder); final PunctuationSpan[] spans = builder.getSpans(0, builder.length(), PunctuationSpan.class); assertEquals(2, spans.length); final PunctuationSpan first = spans[0]; assertEquals(0, builder.getSpanStart(first)); assertEquals(2, builder.getSpanEnd(first)); final PunctuationSpan second = spans[1]; assertEquals(6, builder.getSpanStart(second)); assertEquals(8, builder.getSpanEnd(second)); }
Example #6
Source File: InlineParserActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void disableHtmlSanitize() { final String md = "# Html <b>disabled</b>\n\n" + "<em>emphasis <strong>strong</strong>\n\n" + "<p>paragraph <img src='hey.jpg' /></p>\n\n" + "<test></test>\n\n" + "<test>"; final Markwon markwon = Markwon.builder(this) .usePlugin(new AbstractMarkwonPlugin() { @NonNull @Override public String processMarkdown(@NonNull String markdown) { return markdown .replaceAll("<", "<") .replaceAll(">", ">"); } }) .build(); markwon.setMarkdown(textView, md); }
Example #7
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void letterOrderedList() { // bullet list nested in ordered list renders letters instead of bullets final String md = "" + "1. Hello there!\n" + "1. And here is how:\n" + " - First\n" + " - Second\n" + " - Third\n" + " 1. And first here\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(new BulletListIsOrderedWithLettersWhenNestedPlugin()) .build(); markwon.setMarkdown(textView, md); }
Example #8
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void tableOfContents() { final String lorem = getString(R.string.lorem); final String md = "" + "# First\n" + "" + lorem + "\n\n" + "# Second\n" + "" + lorem + "\n\n" + "## Second level\n\n" + "" + lorem + "\n\n" + "### Level 3\n\n" + "" + lorem + "\n\n" + "# First again\n" + "" + lorem + "\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(new TableOfContentsPlugin()) .usePlugin(new AnchorHeadingPlugin((view, top) -> scrollView.smoothScrollTo(0, top))) .build(); markwon.setMarkdown(textView, md); }
Example #9
Source File: LatexActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void renderWithBlocksAndInlines(@NonNull String markdown) { final float textSize = textView.getTextSize(); final Resources r = getResources(); final Markwon markwon = Markwon.builder(this) // NB! `MarkwonInlineParserPlugin` is required in order to parse inlines .usePlugin(MarkwonInlineParserPlugin.create()) .usePlugin(JLatexMathPlugin.create(textSize, textSize * 1.25F, builder -> { // Important thing to do is to enable inlines (by default disabled) builder.inlinesEnabled(true); builder.theme() .inlineBackgroundProvider(() -> new ColorDrawable(0x1000ff00)) .blockBackgroundProvider(() -> new ColorDrawable(0x10ff0000)) .blockPadding(JLatexMathTheme.Padding.symmetric( r.getDimensionPixelSize(R.dimen.latex_block_padding_vertical), r.getDimensionPixelSize(R.dimen.latex_block_padding_horizontal) )); })) .build(); markwon.setMarkdown(textView, markdown); }
Example #10
Source File: PrecomputedActivity.java From Markwon with Apache License 2.0 | 6 votes |
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_text_view); final Markwon markwon = Markwon.builder(this) // please note that precomputedTextCompat is no-op on devices lower than L (21) .textSetter(PrecomputedTextSetterCompat.create(Executors.newCachedThreadPool())) .build(); final TextView textView = findViewById(R.id.text_view); final String markdown = "# Hello!\n\n" + "This _displays_ how to implement and use `PrecomputedTextCompat` with the **Markwon**\n\n" + "> consider using PrecomputedText only if your markdown content is large enough\n> \n" + "> **please note** that it works badly with `markwon-recycler` due to asynchronous nature"; // please note that _sometimes_ (if done without `post` here) further `textView.post` // (that is used in PrecomputedTextSetterCompat to deliver result to main-thread) won't be called // making the result of pre-computation absent and text-view clear (no text) textView.post(() -> markwon.setMarkdown(textView, markdown)); }
Example #11
Source File: PrecomputedFutureActivity.java From Markwon with Apache License 2.0 | 6 votes |
@Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_recycler); final Markwon markwon = Markwon.builder(this) .textSetter(PrecomputedFutureTextSetterCompat.create()) .build(); // create MarkwonAdapter and register two blocks that will be rendered differently final MarkwonAdapter adapter = MarkwonAdapter.builder(R.layout.adapter_appcompat_default_entry, R.id.text) .build(); final RecyclerView recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setHasFixedSize(true); recyclerView.setAdapter(adapter); adapter.setMarkdown(markwon, loadReadMe(this)); // please note that we should notify updates (adapter doesn't do it implicitly) adapter.notifyDataSetChanged(); }
Example #12
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void customize() { final String md = "" + "| HEADER | HEADER | HEADER |\n" + "|:----:|:----:|:----:|\n" + "| 测试 | 测试 | 测试 |\n" + "| 测试 | 测试 | 测测测12345试测试测试 |\n" + "| 测试 | 测试 | 123445 |\n" + "| 测试 | 测试 | (650) 555-1212 |\n" + "| 测试 | 测试 | [link](#) |\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(TablePlugin.create(builder -> { final Dip dip = Dip.create(this); builder .tableBorderWidth(dip.toPx(2)) .tableBorderColor(Color.YELLOW) .tableCellPadding(dip.toPx(4)) .tableHeaderRowBackgroundColor(ColorUtils.applyAlpha(Color.RED, 80)) .tableEvenRowBackgroundColor(ColorUtils.applyAlpha(Color.GREEN, 80)) .tableOddRowBackgroundColor(ColorUtils.applyAlpha(Color.BLUE, 80)); })) .build(); markwon.setMarkdown(textView, md); }
Example #13
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void tableAndLinkify() { final String md = "" + "| HEADER | HEADER | HEADER |\n" + "|:----:|:----:|:----:|\n" + "| 测试 | 测试 | 测试 |\n" + "| 测试 | 测试 | 测测测12345试测试测试 |\n" + "| 测试 | 测试 | 123445 |\n" + "| 测试 | 测试 | (650) 555-1212 |\n" + "| 测试 | 测试 | [link](#) |\n" + "\n" + "测试\n" + "\n" + "[link link](https://link.link)"; final Markwon markwon = Markwon.builder(this) .usePlugin(LinkifyPlugin.create()) .usePlugin(TablePlugin.create(this)) .build(); markwon.setMarkdown(textView, md); }
Example #14
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void withLatex() { String latex = "\\begin{array}{cc}"; latex += "\\fbox{\\text{A framed box with \\textdbend}}&\\shadowbox{\\text{A shadowed box}}\\cr"; latex += "\\doublebox{\\text{A double framed box}}&\\ovalbox{\\text{An oval framed box}}\\cr"; latex += "\\end{array}"; final String md = "" + "| HEADER | HEADER |\n" + "|:----:|:----:|\n" + "|  | Build |\n" + "| Stable |  |\n" + "| BIG | $$" + latex + "$$ |\n" + "\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(MarkwonInlineParserPlugin.create()) .usePlugin(ImagesPlugin.create()) .usePlugin(JLatexMathPlugin.create(textView.getTextSize(), builder -> builder.inlinesEnabled(true))) .usePlugin(TablePlugin.create(this)) .build(); markwon.setMarkdown(textView, md); }
Example #15
Source File: EditorActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void plugin_require() { // usage of plugin from other plugins final Markwon markwon = Markwon.builder(this) .usePlugin(MarkwonInlineParserPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(MarkwonInlineParserPlugin.class) .factoryBuilder() .excludeInlineProcessor(HtmlInlineProcessor.class); } }) .build(); editText.setMovementMethod(LinkMovementMethod.getInstance()); final MarkwonEditor editor = MarkwonEditor.create(markwon); editText.addTextChangedListener(MarkwonEditorTextWatcher.withPreRender( editor, Executors.newSingleThreadExecutor(), editText)); }
Example #16
Source File: EditorActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void plugin_no_defaults() { // a plugin with no defaults registered final Markwon markwon = Markwon.builder(this) .usePlugin(MarkwonInlineParserPlugin.create(MarkwonInlineParser.factoryBuilderNoDefaults())) // .usePlugin(MarkwonInlineParserPlugin.create(MarkwonInlineParser.factoryBuilderNoDefaults(), factoryBuilder -> { // // if anything, they can be included here //// factoryBuilder.includeDefaults() // })) .build(); final MarkwonEditor editor = MarkwonEditor.create(markwon); editText.addTextChangedListener(MarkwonEditorTextWatcher.withPreRender( editor, Executors.newSingleThreadExecutor(), editText)); }
Example #17
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void align() { final String md = "" + "<align center>We are centered</align>\n" + "\n" + "<align end>We are at the end</align>\n" + "\n" + "<align>We should be at the start</align>\n" + "\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(HtmlPlugin.class, htmlPlugin -> htmlPlugin .addHandler(new AlignTagHandler())); } }) .build(); markwon.setMarkdown(textView, md); }
Example #18
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void randomCharSize() { final String md = "" + "<random-char-size>\n" + "This message should have a jumpy feeling because of different sizes of characters\n" + "</random-char-size>\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(HtmlPlugin.class, htmlPlugin -> htmlPlugin .addHandler(new RandomCharSize(new Random(42L), textView.getTextSize()))); } }) .build(); markwon.setMarkdown(textView, md); }
Example #19
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void enhance() { final String md = "" + "<enhance start=\"5\" end=\"12\">This is text that must be enhanced, at least a part of it</enhance>"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(HtmlPlugin.class, htmlPlugin -> htmlPlugin .addHandler(new EnhanceTagHandler((int) (textView.getTextSize() * 2 + .05F)))); } }) .build(); markwon.setMarkdown(textView, md); }
Example #20
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void image() { // treat unclosed/void `img` tag as HTML inline final String md = "" + "## Try CommonMark\n" + "\n" + "Markwon IMG:\n" + "\n" + "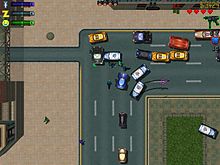\n" + "\n" + "New lines...\n" + "\n" + "HTML IMG:\n" + "\n" + "<img src=\"https://upload.wikimedia.org/wikipedia/it/thumb/c/c5/GTA_2.JPG/220px-GTA_2.JPG\"></img>\n" + "\n" + "New lines\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(ImagesPlugin.create()) .usePlugin(HtmlPlugin.create()) .build(); markwon.setMarkdown(textView, md); }
Example #21
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void withImages() { final String md = "" + "| HEADER | HEADER |\n" + "|:----:|:----:|\n" + "|  | Build |\n" + "| Stable |  |\n" + "| BIG | 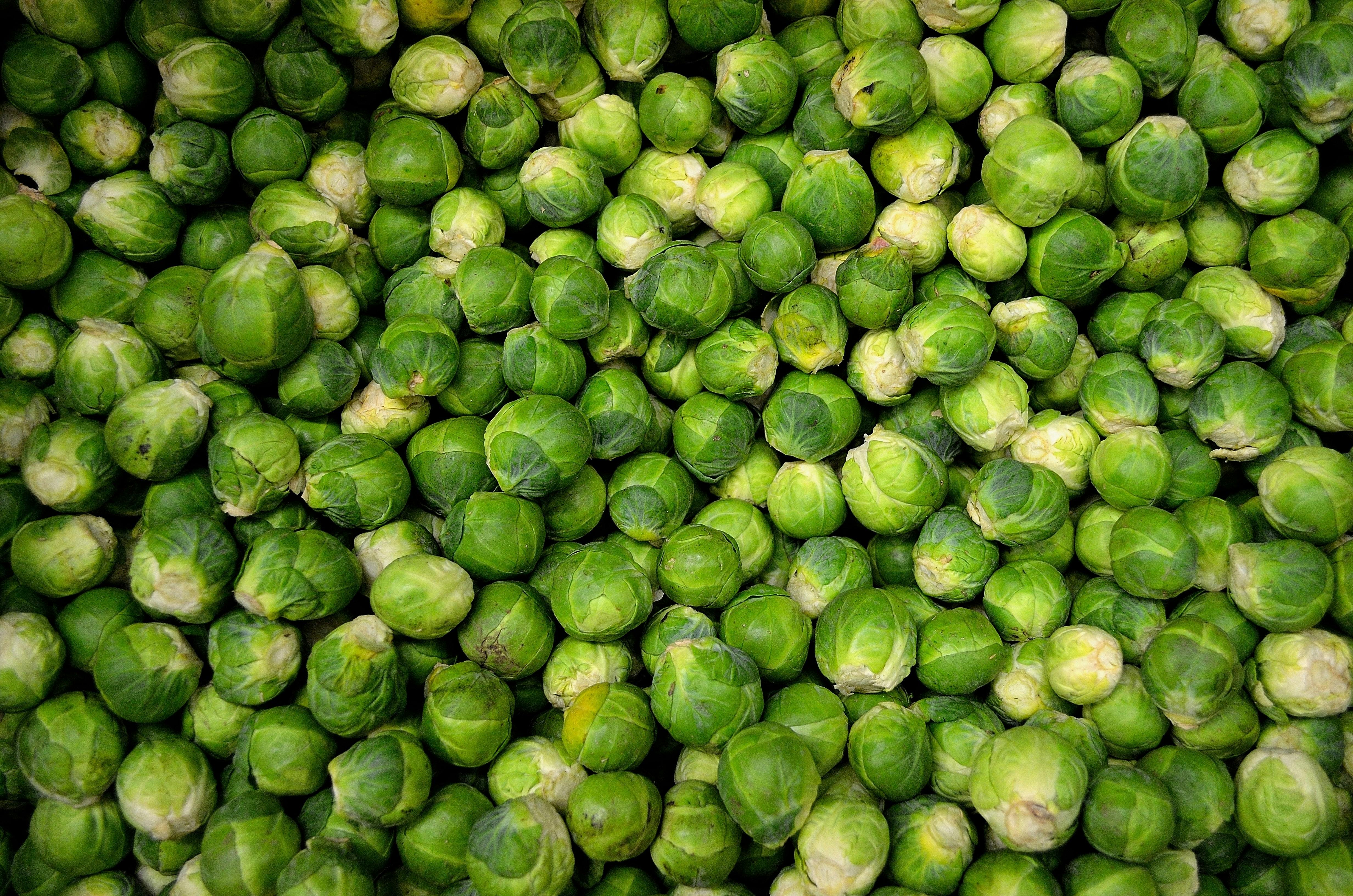 |\n" + "\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(ImagesPlugin.create()) .usePlugin(TablePlugin.create(this)) .build(); markwon.setMarkdown(textView, md); }
Example #22
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void emptyTagReplacement() { final String md = "" + "<empty></empty> the `<empty></empty>` is replaced?"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create(plugin -> { plugin.emptyTagReplacement(new HtmlEmptyTagReplacement() { @Nullable @Override public String replace(@NonNull HtmlTag tag) { if ("empty".equals(tag.name())) { return "REPLACED_EMPTY_WITH_IT"; } return super.replace(tag); } }); })) .build(); markwon.setMarkdown(textView, md); }
Example #23
Source File: CustomExtensionActivity.java From Markwon with Apache License 2.0 | 6 votes |
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_text_view); final TextView textView = findViewById(R.id.text_view); // note that we haven't registered CorePlugin, as it's the only one that can be // implicitly deducted and added automatically. All other plugins require explicit // `usePlugin` call final Markwon markwon = Markwon.builder(this) // try commenting out this line to see runtime dependency resolution // .usePlugin(ImagesPlugin.create(this)) .usePlugin(IconPlugin.create(IconSpanProvider.create(this, 0))) .build(); markwon.setMarkdown(textView, getString(R.string.input)); }
Example #24
Source File: ListMessageAdapter.java From android with MIT License | 6 votes |
ListMessageAdapter( Context context, Settings settings, Picasso picasso, List<MessageWithImage> items, Delete delete) { super(); this.context = context; this.settings = settings; this.picasso = picasso; this.items = items; this.delete = delete; this.markwon = Markwon.builder(context) .usePlugin(CorePlugin.create()) .usePlugin(MovementMethodPlugin.create()) .usePlugin(PicassoImagesPlugin.create(picasso)) .usePlugin(TablePlugin.create(context)) .build(); }
Example #25
Source File: SimpleExtActivity.java From Markwon with Apache License 2.0 | 6 votes |
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_text_view); final TextView textView = findViewById(R.id.text_view); final Markwon markwon = Markwon.builder(this) .usePlugin(SimpleExtPlugin.create(plugin -> plugin // +sometext+ .addExtension(1, '+', (_1, _2) -> new EmphasisSpan()) // ??sometext?? .addExtension(2, '?', (_1, _2) -> new StrongEmphasisSpan()) // @@sometext$$ .addExtension(2, '@', '$', (_1, _2) -> new ForegroundColorSpan(Color.RED)))) .build(); final String markdown = "# SimpleExt\n" + "\n" + "+let's start with `+`, ??then we can use this, and finally @@this$$??+"; markwon.setMarkdown(textView, markdown); }
Example #26
Source File: EditorActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void simple_process() { // Process highlight in-place (right after text has changed) // obtain Markwon instance final Markwon markwon = Markwon.create(this); // create editor final MarkwonEditor editor = MarkwonEditor.create(markwon); // set edit listener editText.addTextChangedListener(MarkwonEditorTextWatcher.withProcess(editor)); }
Example #27
Source File: EditorActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void additional_plugins() { // As highlight works based on text-diff, everything that is present in input, // but missing in resulting markdown is considered to be punctuation, this is why // additional plugins do not need special handling final Markwon markwon = Markwon.builder(this) .usePlugin(StrikethroughPlugin.create()) .build(); final MarkwonEditor editor = MarkwonEditor.create(markwon); editText.addTextChangedListener(MarkwonEditorTextWatcher.withProcess(editor)); }
Example #28
Source File: EditorActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void heading() { final Markwon markwon = Markwon.create(this); final MarkwonEditor editor = MarkwonEditor.builder(markwon) .useEditHandler(new HeadingEditHandler()) .build(); editText.addTextChangedListener(MarkwonEditorTextWatcher.withPreRender( editor, Executors.newSingleThreadExecutor(), editText)); }
Example #29
Source File: EditorActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void simple_pre_render() { // Process highlight in background thread final Markwon markwon = Markwon.create(this); final MarkwonEditor editor = MarkwonEditor.create(markwon); editText.addTextChangedListener(MarkwonEditorTextWatcher.withPreRender( editor, Executors.newCachedThreadPool(), editText)); }
Example #30
Source File: TaskListActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void customDrawableResources() { // drawable **must** be stateful final Drawable drawable = Objects.requireNonNull( ContextCompat.getDrawable(this, R.drawable.custom_task_list)); final Markwon markwon = Markwon.builder(this) .usePlugin(TaskListPlugin.create(drawable)) .build(); markwon.setMarkdown(textView, MD); }